SAP ABAP (Advanced Business Application Programming) plays a critical role in data processing within SAP systems. One of its key concepts is the internal table, a powerful structure used to handle and manipulate data dynamically during program execution. In this article, we will explore SAP internal tables, their types, common operations, and the concept of work area in detail.
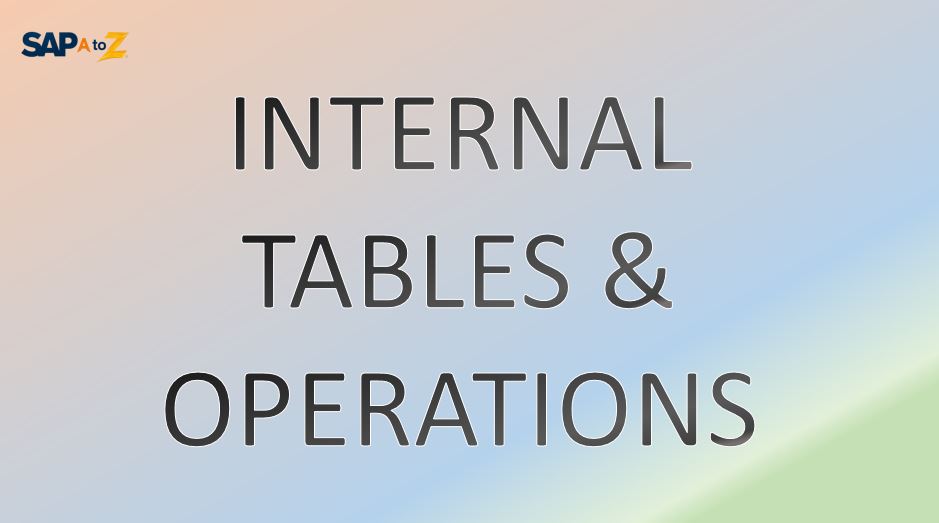
What is an Internal Table in SAP?
An internal table in SAP ABAP is a temporary storage structure used to store and process data during program execution. Unlike database tables, internal tables reside in application server memory and are primarily used for structured data processing within an ABAP program.
Internal tables are particularly useful for handling large datasets retrieved from database tables, processing data before updating the database, and exchanging data between different parts of a program.
Types of Internal Tables in SAP
SAP ABAP provides three main types of internal tables, each serving different purposes:
1. Standard Table
A standard table is the most common type of internal table. It has a linear index and allows access to records sequentially or via an index. Searching for records in a standard table without an index requires a linear search, which may impact performance for large datasets.
Example of Standard Table Declaration
DATA: lt_materials TYPE TABLE OF mara.
Here, lt_materials
is an internal table that holds data from the MARA
(Material Master) table.
2. Sorted Table
A sorted table is an internal table that maintains records in a sorted order based on the primary key. It allows binary searches, making data retrieval faster than in a standard table.
Example of Sorted Table Declaration
DATA: lt_sorted_materials TYPE SORTED TABLE OF mara WITH UNIQUE KEY matnr.
Here, lt_sorted_materials
stores material data sorted by the MATNR
field.
3. Hashed Table
A hashed table uses a hashing algorithm to store and retrieve records quickly. This type of table is ideal for scenarios where frequent key-based access is required, but it does not support index-based access like standard or sorted tables.
Example of Hashed Table Declaration
DATA: lt_hashed_materials TYPE HASHED TABLE OF mara WITH UNIQUE KEY matnr.
This declaration creates a hashed table where MATNR
is the unique key, ensuring fast key-based access.
Internal Table Operations
ABAP provides various operations to handle and manipulate internal tables efficiently. Below are some of the most common operations:
1. Populating an Internal Table
Data can be inserted into an internal table using the SELECT
statement or manually with APPEND
and INSERT
.
Fetching Data from a Database Table
SELECT * FROM mara INTO TABLE lt_materials WHERE mtart = 'RAW'.
This statement fetches all materials of type ‘RAW’ and stores them in lt_materials
.
Appending Data Manually
DATA: wa_material TYPE mara.
wa_material-matnr = '1001'.
wa_material-mtart = 'ROH'.
APPEND wa_material TO lt_materials.
This manually appends a record to the internal table.
2. Reading Data from an Internal Table
Data retrieval can be done using READ TABLE
or looping through the internal table.
Using READ TABLE
DATA: wa_material TYPE mara.
READ TABLE lt_materials INTO wa_material WITH KEY matnr = '1001'.
IF sy-subrc = 0.
WRITE: 'Material found:', wa_material-mtart.
ENDIF.
This searches for a material with MATNR = '1001'
and stores the result in wa_material
.
3. Modifying Data in an Internal Table
Data in an internal table can be modified using MODIFY
.
Updating an Entry
READ TABLE lt_materials INTO wa_material WITH KEY matnr = '1001'.
IF sy-subrc = 0.
wa_material-mtart = 'FERT'.
MODIFY lt_materials FROM wa_material.
ENDIF.
This updates the MTART
field of the material 1001
to FERT
.
4. Deleting Data from an Internal Table
Records can be removed using DELETE
.
Deleting a Record by Key
DELETE lt_materials WHERE matnr = '1001'.
This removes the record where MATNR = '1001'
.
5. Sorting an Internal Table
Sorting helps arrange data in ascending or descending order.
SORT lt_materials BY matnr ASCENDING.
This sorts lt_materials
by MATNR
in ascending order.
Understanding Workarea in SAP
A workarea is a temporary variable that holds a single row of an internal table. It is used for processing records one at a time before inserting, reading, modifying, or deleting data in an internal table.
Declaring and Using a Workarea
DATA: wa_material TYPE mara.
LOOP AT lt_materials INTO wa_material.
WRITE: wa_material-matnr, wa_material-mtart.
ENDLOOP.
Here, wa_material
holds each row of lt_materials
one at a time during the loop execution.
Best Practices for Working with Internal Tables
- Choose the Right Table Type: Use hashed tables for quick key-based access, sorted tables for sorted data, and standard tables for general storage.
- Minimize Data Load: Fetch only required fields instead of using
SELECT *
to improve performance. - Use Proper Indexing: Define keys correctly in sorted and hashed tables for efficient retrieval.
- Optimize Search Operations: Use
READ TABLE ... WITH KEY
and binary search for better performance. - Avoid Unnecessary Loops: Use table operations like
MODIFY
,DELETE
, andSORT
instead of unnecessary loops.
Know more on SAP
Pingback: Mastering Core ABAP: 12 Key Concepts for SAP Development