SAP modularization helps in writing clean, reusable, and well-structured ABAP programs. It reduces redundancy and enhances readability. Developers can break large programs into smaller, manageable parts. This also improves debugging and maintenance. Let’s explore different modularization techniques in SAP ABAP.
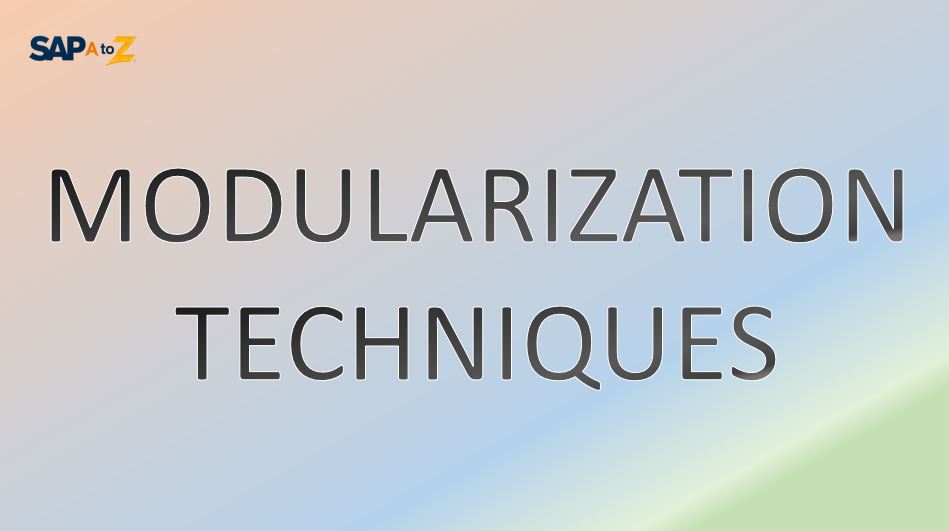
1. Why Modularization is Important in SAP?
When we write long programs, they become complex. Maintaining them is difficult. Modularization helps by splitting a program into smaller parts. It offers several benefits:
- Reduces duplicate code.
- Increases readability.
- Improves debugging and maintenance.
- Enhances reusability.
- Saves time and effort.
- Increases performance by reducing redundant processing.
SAP provides different modularization techniques. They help in structuring the code better.
2. Types of Modularization Techniques in SAP
SAP supports various modularization techniques in ABAP:
- Includes
- Subroutines (FORM…END FORM)
- Function Modules
- Methods (Object-Oriented Approach)
- Macros
- Local and Global Classes
Let’s understand each one in detail.
2.1 Includes
Includes are used to store reusable code in separate files. These files can be used in multiple programs. Instead of writing the same code again, we call the include file.
Example:
INCLUDE zprogram_include.
Here, zprogram_include
is an include file containing reusable ABAP code. We can place SELECT statements, data declarations, or subroutines inside it.
Advantages:
- Helps in breaking long programs.
- Improves code readability.
- Easy to maintain.
2.2 Subroutines (FORM…END FORM)
Subroutines, also known as Forms, allow code reusability within a program. We define them using FORM
and ENDFORM
.
Example:
FORM display_message.
WRITE: 'Hello, SAP!'.
ENDFORM.
To call the subroutine, use:
PERFORM display_message.
Advantages:
- Reduces duplicate code.
- Improves modularity within a program.
- Makes debugging easier.
2.3 Function Modules
Function modules are stored in Function Groups. They allow code reuse across different programs. They are created using SE37 transaction.
Example:
CALL FUNCTION 'Z_CALCULATE_TOTAL'
EXPORTING
value1 = lv_num1
value2 = lv_num2
IMPORTING
result = lv_result.
Here, Z_CALCULATE_TOTAL
is a function module that performs calculations.
Advantages:
- Used globally across programs.
- Supports exception handling.
- Increases efficiency.
2.4 Methods (Object-Oriented Approach)
Methods are part of Classes in Object-Oriented Programming (OOP). They provide better encapsulation and flexibility.
Example:
CLASS zcl_math DEFINITION.
PUBLIC SECTION.
METHODS add IMPORTING num1 TYPE i num2 TYPE i EXPORTING result TYPE i.
ENDCLASS.
CLASS zcl_math IMPLEMENTATION.
METHOD add.
result = num1 + num2.
ENDMETHOD.
ENDCLASS.
To call the method:
DATA: obj TYPE REF TO zcl_math,
lv_result TYPE i.
CREATE OBJECT obj.
CALL METHOD obj->add
EXPORTING num1 = 5 num2 = 10
IMPORTING result = lv_result.
Advantages:
- Supports encapsulation and reusability.
- Used in modern ABAP development.
- Makes the code more organized.
2.5 Macros
Macros are used to define reusable code blocks in a program. They are defined using DEFINE
and END-OF-DEFINITION
keywords.
Example:
DEFINE write_message.
WRITE: 'This is a macro!'.
END-OF-DEFINITION.
write_message.
Advantages:
- Used for repetitive small tasks.
- Reduces code writing effort.
- Improves performance.
2.6 Local and Global Classes
Classes in SAP ABAP are used in Object-Oriented Programming to create reusable objects.
- Local Classes: Used within a single program.
- Global Classes: Created in SE24 and used across multiple programs.
Using classes provides better structuring and reusability in ABAP programs.
3. Best Practices for SAP Modularization
- Use meaningful names for subroutines, function modules, and methods.
- Avoid unnecessary duplication of code.
- Keep the modularized code simple and focused.
- Use exception handling in function modules.
- Follow naming conventions for better understanding.
- Use function modules for reusable logic.
- Prefer methods and classes for new developments.
- Avoid deeply nested includes to maintain readability.
SAP modularization helps in writing structured and maintainable code. Includes, subroutines, function modules, methods, macros, and classes improve reusability. Choosing the right technique depends on the requirement. By following best practices, developers can ensure efficient ABAP programming.
Modularization makes SAP development easier and saves time. Start using these techniques in your ABAP programs today!
Pingback: Mastering Core ABAP: 12 Key Concepts for SAP Development