Introduction to Object Oriented ABAP
SAP ABAP (Advanced Business Application Programming) has evolved significantly over the years. Traditional ABAP relied on procedural programming. However, SAP introduced Object-Oriented ABAP (OOABAP) to bring modern programming concepts into the SAP environment.
OOABAP allows developers to write modular, reusable, and maintainable code. It follows the principles of Object-Oriented Programming (OOP), such as encapsulation, inheritance, and polymorphism.
In this article, you will learn the fundamentals of Object-Oriented ABAP with examples and best practices.
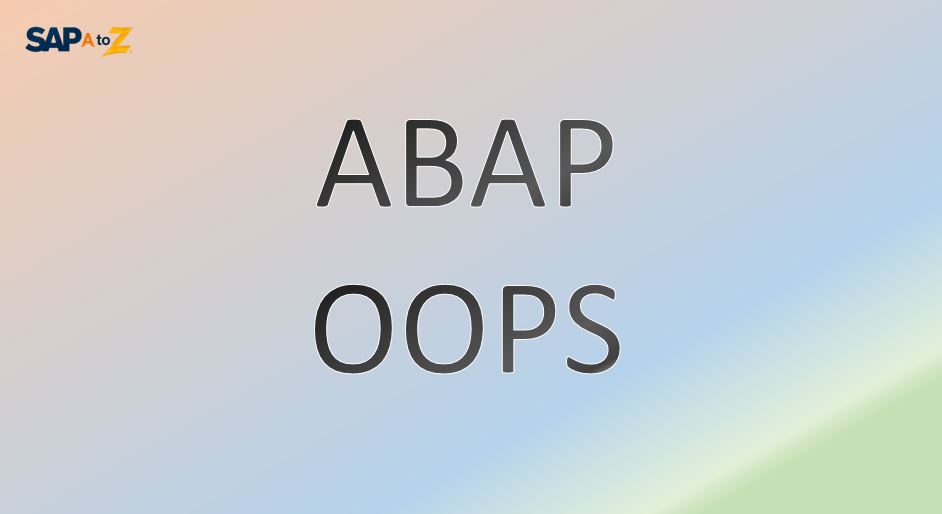
What is Object Oriented ABAP?
Object-Oriented ABAP is an extension of ABAP that enables developers to use OOP concepts. It helps in structuring code better and makes it easier to maintain and scale.
Key OOP concepts in ABAP include:
- Classes and Objects
- Encapsulation
- Inheritance
- Polymorphism
- Interfaces
Advantages of Object Oriented ABAP
- Better Code Reusability – Common functionalities can be reused across multiple programs.
- Improved Maintainability – Structured code is easier to manage and update.
- Encapsulation – Data hiding improves security and reduces errors.
- Flexibility – Enhancements can be made without modifying the original code.
- Scalability – Supports complex applications with structured coding practices.
Understanding Classes and Objects
In OOABAP, a class is a blueprint for creating objects. An object is an instance of a class.
Defining a Class in ABAP
CLASS lcl_vehicle DEFINITION.
PUBLIC SECTION.
DATA: color TYPE string.
METHODS: display_color.
ENDCLASS.
Implementing Methods
CLASS lcl_vehicle IMPLEMENTATION.
METHOD display_color.
WRITE: 'Vehicle color:', color.
ENDMETHOD.
ENDCLASS.
Creating an Object
DATA: lo_car TYPE REF TO lcl_vehicle.
CREATE OBJECT lo_car.
lo_car->color = 'Red'.
lo_car->display_color().
Attributes and Sections in ABAP Classes
A class in ABAP consists of different sections that define visibility and accessibility of attributes and methods.
Types of Class Sections
- Public Section – Accessible from outside the class.
- Protected Section – Accessible only within the class and its subclasses.
- Private Section – Accessible only within the class.
Example of Class Sections
CLASS lcl_example DEFINITION.
PUBLIC SECTION.
DATA: public_attr TYPE string.
METHODS: display_public.
PROTECTED SECTION.
DATA: protected_attr TYPE string.
METHODS: display_protected.
PRIVATE SECTION.
DATA: private_attr TYPE string.
METHODS: display_private.
ENDCLASS.
Encapsulation in ABAP
Encapsulation restricts direct access to some components of an object. It ensures that only required data is accessible.
Using Private and Public Sections
CLASS lcl_person DEFINITION.
PRIVATE SECTION.
DATA name TYPE string.
PUBLIC SECTION.
METHODS: set_name IMPORTING p_name TYPE string,
get_name RETURNING VALUE(r_name) TYPE string.
ENDCLASS.
CLASS lcl_person IMPLEMENTATION.
METHOD set_name.
name = p_name.
ENDMETHOD.
METHOD get_name.
r_name = name.
ENDMETHOD.
ENDCLASS.
Inheritance in OOABAP
Inheritance allows a class to acquire properties and methods of another class.
Example of Inheritance
CLASS lcl_animal DEFINITION.
PUBLIC SECTION.
METHODS: make_sound.
ENDCLASS.
CLASS lcl_dog DEFINITION INHERITING FROM lcl_animal.
PUBLIC SECTION.
METHODS: bark.
ENDCLASS.
CLASS lcl_dog IMPLEMENTATION.
METHOD bark.
WRITE: 'Dog is barking'.
ENDMETHOD.
ENDCLASS.
DATA: lo_dog TYPE REF TO lcl_dog.
CREATE OBJECT lo_dog.
lo_dog->bark().
Polymorphism in ABAP
Polymorphism allows a single interface to be used for different data types.
Example Using Method Overriding
CLASS lcl_animal DEFINITION.
PUBLIC SECTION.
METHODS: make_sound.
ENDCLASS.
CLASS lcl_cat DEFINITION INHERITING FROM lcl_animal.
PUBLIC SECTION.
METHODS: make_sound REDEFINITION.
ENDCLASS.
CLASS lcl_cat IMPLEMENTATION.
METHOD make_sound.
WRITE: 'Cat meows'.
ENDMETHOD.
ENDCLASS.
DATA: lo_cat TYPE REF TO lcl_cat.
CREATE OBJECT lo_cat.
lo_cat->make_sound().
Interfaces in ABAP
An interface defines a set of methods without implementing them.
Example of an Interface
INTERFACE lif_vehicle.
METHODS: start, stop.
ENDINTERFACE.
CLASS lcl_car DEFINITION.
PUBLIC SECTION.
INTERFACES: lif_vehicle.
ENDCLASS.
CLASS lcl_car IMPLEMENTATION.
METHOD lif_vehicle~start.
WRITE: 'Car started'.
ENDMETHOD.
METHOD lif_vehicle~stop.
WRITE: 'Car stopped'.
ENDMETHOD.
ENDCLASS.
Best Practices for Object Oriented ABAP
- Use meaningful class and method names.
- Encapsulate data to avoid direct modification.
- Follow SAP naming conventions.
- Use interfaces to improve flexibility.
- Avoid unnecessary inheritance.
Object-Oriented ABAP enhances traditional ABAP by introducing OOP concepts. It improves code maintainability, reusability, and scalability.
By mastering OOABAP, you can develop efficient and flexible SAP applications. Start using classes, objects, inheritance, and interfaces in your ABAP programs today!
Know more on SAP
Pingback: Mastering Core ABAP: 12 Key Concepts for SAP Development